4 minutes
Helping Someone Learn to Program
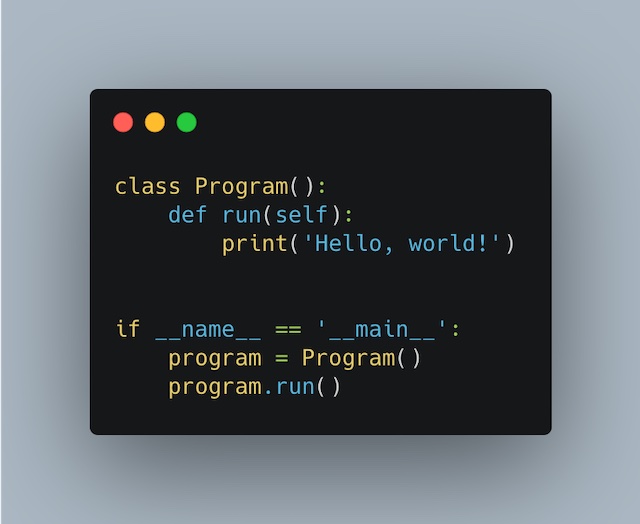
A family member came to me and said that he is interested in learning how to program. Reflecting on my experience learning software in academia I started to think about how I would want to learn programming if I didn’t do so in school.
There are a few key goals I would want to focus on if I was starting out:
- Have a concrete project in mind.
- Learn how to get started quickly.
- Make something useful to put what I am learning into context.
With this in mind I am hoping to point him in a direction that will accelerate his learning and excitement for software. One of the key things with how I am helping is that I don’t have the capacity to be a teacher. I want to get him to a place where he can ask me specific questions. I want our conversations to go from “How can I learn how to program?” to “How do I add text to a file instead of the console?”
Getting Started
Language
Python is a good language to start out with for a ton of reasons. It is easy to get started with, but can become more complex as needed. It can be a bicycle or a rocket ship based on your needs. You can start with a file of just function calls and see something quickly, but you can also build up to object oriented programming and learn more advanced concepts as you need them.
With python you can get started with something as simple as:
print('Hello, world!')
When you start to dig into more advanced features you can migrate to something like:
class Program():
def run(self):
print('Hello, world!')
if __name__ == '__main__':
program = Program()
program.run()
This allows you to get something working fast without needing to understand classes, instances, methods, etc.
As you learn more and want to get into a specific domain the concepts you learn in python will be transferable.
Tools
VS Code is similar to python in the sense that it can grow as you need it. It can start out as a simple text editor, but with plugins you can do things like run a microcontroller simulator. Like Python, VS Code is so popular that if you run into questions you can easily find a ton of online resources to help.
Resources
There are a ton of tutorials, blogs, classes, etc that can help you get started, but my main goal is to get started doing something useful quickly. As such I think Automate The Boring Stuff is one of the best resource you can start with. It wont necessarily teach you how to become a software engineer, but it will teach you how to solve problems with code. It will give you the tools to build useful things to solve problems. Once you work through parts of the book there you should be at a point where you can build out solutions. You will also be able to read some basic source code to be able to start to understand this vast world of software.
Goals
Over 2 - 3 weeks I think there are some concrete things should be done.
- Have a project in mind that you can build up to. Write it down and think of the requirements: What do you want it to do? What data does it need? What data do you need to get out of it?
- Finish Chapter 0-3 of Automate The Boring Stuff.
- Watch lessons 1-11 of the playlist. (these go over chapter 0-3) of the book
Summary
In my experience useful summaries come in the form of questions that can be answered after working through material.
At the end of the couple of weeks spent working towards learning you should be able to answer all of these questions:
- What is a program?
- What is a variable?
- What is a conditional?
- What is a for loop?
- What is a function?
- Explain how to use loops and conditionals to print out the song “99 Bottles of Beer on the Wall”
- Write a program to do this.
- Describe a problem you currently have that you can use programming to solve.
After getting comfortable with these things you should start to build on what you have learned so far to get deeper into programming. The goal is to have a project in mind that you can start working on to apply what you learn. You should also be at a place where you can ask pointed questions and get direction rather than ambiguous questions.
References
As I worked through the best ways to learn how to program these resources help influence my approach.